processObjectTypeParameterOfPugMixin
- Generic technical name
- compoundParameter
- Type
- CompoundParameter
- rawParameter
- Required if
- At least one of "parameterPropertiesSpecification"'s properties is marked as required
- Type
- ParsedJSON_Object
- parameterPropertiesSpecification
- Required
- Yes
- Type
- RawObjectDataProcessor.PropertiesSpecification
- parameterNumber
- Required
- Yes
- Type
- number
- parameterName
- Required
- Yes
- Type
- string
- mixinName
- Required
- Yes
- Type
- string
Validates and processes the object-type parameter of the Pug mixin.
Such functionality should be required when implementing GUI components or other markup fragments using Pug mixins with a large number of customizable properties, wherein some (sometimes all) of them must satisfy certain limitations for generating correct HTML code.
Problems
The HTML preprocessor Pug, like internal JavaScript is not a statically-typed language. When using Pug mixins with parameters, if the specified parameters are invalid (the number of parameters or their types, etc.), there will be no advance notification to the developer. Even if an error occurs during Pug-to-HTML conversion, the cause and location of the error could be unclear, sometimes resulting in unexpected HTML code without any errors, which developers might not notice for a long time. This is especially critical when the mixins are provided by third-party libraries, not written by yourself, including when implementing GUI components via Pug mixins.
The primitive solution to this problem is checking the types of parameters and other properties such as character count or number set using native JavaScript.
mixin Example(foo, bar, baz)
-
if (typeof foo !== "string") {
throw new Error(
`The type of the 1st parameter of the "Example" mixin must be a string. Type found: "${ typeof foo }".`
);
}
if (foo.length < 2) {
throw new Error("1st parameter of the \"Example\" mixin must have at least two characters.");
}
if (typeof bar !== "number") {
throw new Error(
`The type of the 2nd parameter of the "Example" mixin must be a number. Type found: "${ typeof bar }".`
);
}
if (!Number.isInteger(bar)) {
throw new Error("The 2nd parameter of \"Example\" mixin must be an integer.");
}
if (bar < 10) {
throw new Error(
`The 2nd parameter of "Example" has been specified as ${ bar }, while the required minimal value is 10.`
);
}
if (typeof baz !== "boolean") {
throw new Error(
`The type of the 3rd parameter of the "Example" mixin must be a boolean. Type found: ${ typeof baz }".`
);
}
As you can see, validating even three parameters takes a significant part of the code of the Pug mixin, and the error messages are formulaic so, in other mixins, almost the same phrases need to be typed. Now, what if there are not just three parameters, but more? Also, what if there are tens of such mixins? This situation is entirely realistic because, for the average web application, having 20-30 GUI components with flexible settings via plenty of parameters is normal. Thus, manually validating parameters like in the above example would consume a lot of time, mental energy, and lines of code.
Here, the question about a unified mechanism for parameter validation arises. Such a mechanism must check the parameters and their properties. If something is wrong, it should log the details about all violations, (not only the first one). The terminal output must be understandable enough so that users of the Pug mixin can quickly fix the violation themselves without spending time searching for relevant documentation.
The YDF solution
Using the YDEE integration , specifically RawObjectDataProcessor class the function processObjectTypeParameterOfPugMixin has been developed. As it is clear from the function name, this function processes one object-type parameter of the Pug mixin.
The tool for the validation of an arbitrary number of parameters of any type
(as in the +Example(foo, bar, baz)
example) has not
been developed because mixins (same as functions) with a large number
of parameters cause problems in both maintenance and usage.
For example, in the +Example("top", 2, false)
, it is unclear what
semantically means 2
and false
.
Even if the IDE displays hints, in services like GitHub, they
will not be available during code review (and finding a code editor or plugin that displays hints for
the parameters of Pug mixins can be a challenge).
Fortunately, this problem can be easily solved with JavaScript-like Pug syntax by combining the
parameters into a single object type:
mixin Example(compoundParameter)
const { foo, bar, baz } = compoundParameter;
In the above example, using destructuring assignment, all
properties have been extracted to separate constants,
and now they can be used as if they were individual parameters.
When using processObjectTypeParameterOfPugMixin
, you need to destructure
the value that this function returns, not the parameter
compoundParameter
in the above example:
mixin Example(compoundParameter)
const {
foo,
bar,
baz
} = processObjectTypeParameterOfPugMixin({
rawParameter: compoundParameter,
parameterNumber: 1,
parameterName: "compoundParameter",
parameterPropertiesSpecification: {
foo: {
type: String,
required: true,
minimalCharactersCount: 2
},
bar: {
type: Number,
numbersSet: RawObjectDataProcessor.NumbersSets.nonNegativeIngeter,
required: true,
minimalValue: 10
},
baz: {
type: Boolean,
required: true
}
},
mixinName: "Example"
});
As you can see, this function also accepts the sole
parameter of the object type.
The reasons are the same as described above for the Pug mixins.
Among the properties of this parameter is
rawParameter
.
This one accepts the specific parameter of the Pug mixin.
(In the above example, there is only one.)
Another important property, parameterPropertiesSpecification
,
must be defined with the validation rules and, optionally, the processing such as default
value substitutions.
The parameterPropertiesSpecification
property has the
ObjectSubtypes.fixedKeyAndValuePairsObject type.
In fact, it is an associative array-type object whose
keys must match the
keys of the target parameter of the Pug mixin.
The remaining three properties, parameterNumber
,
parameterName
and mixinName
, are required
only for logging; however, they are also important because,
without specifying them, it could be unclear where exactly the issue has occurred.
The Properties of the Sole Parameter
rawParameter
- Required if
- At least one of "parameterPropertiesSpecification"'s properties is marked as required
- Type
- ParsedJSON_Object
The parameter of the Pug mixin in the initial state.
- Here, "raw" means that no manipulations such as default value substitution have been executed yet on this parameter.
- Must be of the object type; otherwise,
the validation will be immediately completed with an error.
The only exception is when all
properties have been specified as optional in
parameterPropertiesSpecification
. In this case,rawParameter
could be of theundefined
type (in other words, be omitted when invoking the mixin). This way,processObjectTypeParameterOfPugMixin
will replace the omitted parameter with the empty object and also substitute the default values if those have been requested for theparameterPropertiesSpecification
parameter.
mixin Example(compoundParameter)
const {
foo,
bar
} = processObjectTypeParameterOfPugMixin({
rawParameter: compoundParameter,
parameterNumber: 1,
parameterName: "compoundParameter",
parameterPropertiesSpecification: {
foo: {
type: String,
required: false,
minimalCharactersCount: 2
},
bar: {
type: Number,
numbersSet: RawObjectDataProcessor.NumbersSets.nonNegativeIngeter,
defaultValue: 10
}
},
mixinName: "Example"
});
parameterPropertiesSpecification
above, the
foo
property is optional, and the
bar
property has the default value.
This means that the = {}
in
mixin Example(compoundParameter = {})
can be
omitted because
processObjectTypeParameterOfPugMixin
will provide an empty
object.
parameterPropertiesSpecification
- Required
- Yes
- Type
- RawObjectDataProcessor.PropertiesSpecification
The rules of the validation and processing of the target parameter of the Pug mixin in ObjectSubtypes.fixedKeyAndValuePairsObject format. In fact, it is an associative array-type object, in which the keys must match the keys of the target parameter of the Pug mixin.
There are various options that you can select as the values of the
ObjectSubtypes.fixedKeyAndValuePairsObject
associative array,
but for each property of the mixin, you need to specify
at least the type and requirement (property
with "required"
name.
If you want to provide the default value, specify defaultValue
property instead).
Refer to the
documentation for RawObjectDataProcessor for details.
Unfortunately, since you are forced to use JavaScript instead of
TypeScript when using Pug, it is easy for you to make
mistakes when specifying the validation rules, which could lead to incorrect execution of the
function, and it could take you some time to notice and locate the mistake.
However, it does not devalue the usage of
parameterPropertiesSpecification
, because the presence of the validation is
better than absence, and also with parameterPropertiesSpecification
, the routine
code volume will be significantly less. (In fact, we need to specify only what to validate and
under which rules to validate without caring about the implementation of the validation.)
To reduce the probability of a mistake when specifying the validation rules, you can define the
variable of the ObjectSubtypes.fixedKeyAndValuePairsObject type in the
TypeScript file and define all desired properties there, when pasting the value to the
Pug code.
The Logging Properties — parameterNumber
, parameterName
, mixinName
- Required
- Yes
- Type
- number
- Required
- Yes
- Type
- string
- Required
- Yes
- Type
- string
These properties are used exclusively for logging any validation errors.
The number of the mixin parameter — parameterNumber
— must be a natural number; the other two properties
must be strings.
Here is an example of the console output fragment for { parameterNumber: 1 }
,
{ parameterName: "properties" }
, and
{ mixinName: "Badge--YDF" }
:
4| mixin Badge--YDF(properties) 5| > 6| - 7| 8| const { 9| Invalid parameter value Object-type parameter No. 1 (named as "properties") of the Pug mixin "Badge--YDF" has one or more invalid properties: (...)
Quick input in IntelliJ IDEA family of IDEs
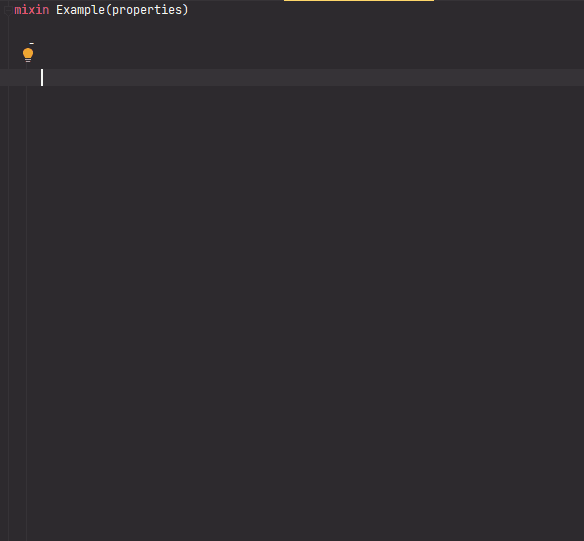
Using the functionality of Live templates in the IntelliJ IDEA family IDEs allows you to input code such as the function invocation expression quickly. To get the Live templates of the YDF library, installation of the official plugin of this library is required.
Steps for using the Live templates
If you have not used Live templates before, do not worry if the instructions below are too complicated. Once you have developed the habit of using Live templates (similar to the habit of using keyboard shortcuts), the following operations will take a matter of seconds.
Before using the Live template, you need to declare the Pug-mixin and specify the parameter(s). You also need to prepare the JavaScript-block inside the mixin body because the following manipulations must be executed inside this block.
- Begin to input the name of the function
processObjectTypeParameterOfPugMixin
. With the current support of the Pug language, most likely, the IDE will not know about the existence of the functionprocessObjectTypeParameterOfPugMixin
if it is not among the project files (excluding dependencies innode_modules
). Therefore, the IDE will offer you only one autocompletion variant. Nonetheless, if two autocompletion variants have appeared — with the letter in the center of the circle and with the cliché, then choose the second variant. - Fill in the property
rawParameter
with a reference to the desired parameter of the mixin. Initially, theproperties
name will be suggested; however, your parameter of the Pug mixin may have a different name. When you are done, press Enter. - Fill in the
parameterNumber
property with the number of parameters of the Pug mixin. You will be suggested to select the parameter number from 1 to 3 in the dropdown list; therefore, if you have between 1 and 3 parameters, then choose the desired number using the arrow keys and press Enter. If you have 4 or more parameters, then press Esc to close the dropdown list, input the desired number, then press Enter. - Fill in the
parameterName
property with the name of the parameter of the Pug mixin. - Fill in the
parameterPropertiesSpecification
property of the object of theObjectSubtypes.fixedKeyAndValuePairsObject
type. To reduce the probability of a mistake, it is recommended that you create a variable of this type in the TypeScript file and copy the value from there. Also, of course, you can input the code directly. - Fill in the
mixinName
property with the name of the Pug mixin.